
State Management in React: React Context and Redux
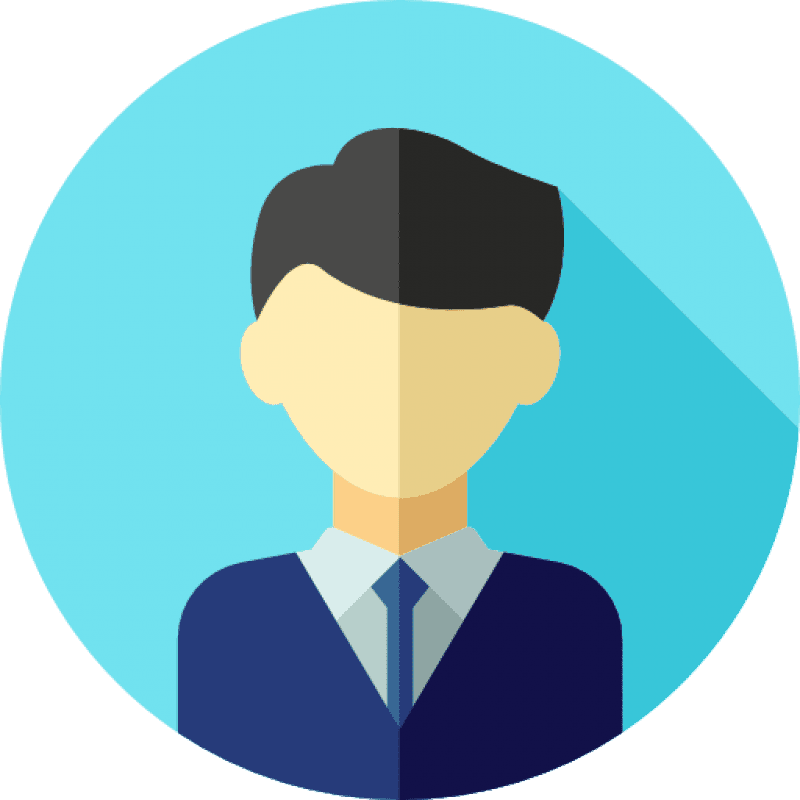
mohamad
Published 21/11/2024 - Last Updated 22/11/2024
#ReactJS#Redux
As web applications become more complex, managing state—the data that changes over time—is one of the most critical challenges developers face. In a React application, the "state" refers to the data that determines how the application looks and behaves. As the application grows, efficiently handling this state becomes increasingly important to ensure your app remains maintainable, performant, and scalable.
In this blog, we’ll explore the concept of state management and dive into two powerful tools for managing state in React applications: React Context and Redux. By the end of this post, you'll have a solid understanding of both tools and when to use them in your projects.
What is State Management?
Before we dive into specific tools, let’s take a moment to understand what we mean by state management.
In a React app, each component has its own local state. This state is typically used to store data that affects that particular component, such as form inputs, visibility of elements, or loading indicators. For smaller applications, managing state at the component level might be sufficient. However, as your application grows and components need to share data or respond to complex user interactions, it becomes increasingly difficult to manage state solely in individual components.
This is where state management comes in. State management refers to the approach or tools used to manage the state of the entire application. The goal is to ensure that all parts of your app can access, update, and react to state changes in a way that is efficient, predictable, and scalable.
The Need for State Management in React
In React, you can manage state in several ways, but when your app becomes more complex—i.e., when multiple components need access to the same data, or when the state needs to be updated from many places—managing state becomes harder. That’s where tools like React Context and Redux come into play.
Prop Drilling
Prop drilling is a term used in React to describe the process of passing data from a part of a component tree to another part by going through other components that do not necessarily need the data, but only pass it down the tree.
To avoid prop drilling, you can use React’s Context API or state management libraries like Redux which allow you to access the state from anywhere in your component tree without having to pass it down through every level.
React Context: A Simple Solution for Prop Drilling
React Context is a built-in tool that allows you to share state across your entire application, without having to pass props down manually through each level of your component tree (a process known as prop drilling).
Context API is used to pass global variables anywhere in the code. It helps when there is a need for sharing state between a lot of nested components. It is light in weight and easier to use, to create a context just need to call React.createContext(). It eliminates the need to install other dependencies or third-party libraries like redux for state management. It has two properties Provider and Consumer.
A provider is used to provide context to the whole application whereas a consumer consume the context provided by nearest provider. In other words The Provider acts as a parent it passes the state to its children whereas the Consumer uses the state that has been passed.
React Context is great for smaller apps or for managing data that doesn’t change frequently, such as user authentication state or theme settings. However, as your application becomes more complex, managing state with Context alone can lead to performance issues, especially when the state needs to be updated frequently or by many components. This is where Redux comes in.
Redux: A More Scalable State Management Solution
Redux is a more robust state management library that provides a centralized store for the entire application's state. Unlike React Context, Redux helps you manage and update state in a predictable, maintainable way, especially when working with large-scale applications that require complex state interactions.
Redux introduces the concept of a single source of truth: all application state is stored in a central store. To update the state, you dispatch actions, which are plain JavaScript objects that describe a state change. These actions are processed by reducers, which are functions that update the state based on the action type.
React-redux is a state management tool which makes it easier to pass these states from one component to another irrespective of their position in the component tree and hence prevents the complexity of the application.
They are several benfits of using react-redux such as:
- It provides centralized state management i.e. a single store for whole application
- It optimizes performance as it prevents re-rendering of component
- Makes the process of debugging easier
- Since it offers persistent state management therefore storing data for long times become easier
How Redux Works
Here’s an overview of how Redux manages state:
- Store The store holds the entire state of your application in a single object. It acts as a centralized repository for all your app’s data.
- Actions
Actions are plain objects that describe an event or user interaction (e.g.,
ADD_ITEM
,REMOVE_ITEM
). Actions don’t directly change the state; they simply describe the action. - Reducers Reducers are pure functions that receive the current state and an action, then return a new state based on the action type. They specify how the state should change in response to each action.
- Dispatching Actions To change the state, you dispatch an action, which triggers the corresponding reducer to update the store.
When to Use React Context vs. Redux?
While both React Context and Redux are used for managing state in React, they serve different purposes and are suited for different types of applications:
- React Context is best for simpler, smaller applications where you need to share state across components without complex state management. It’s also great for non-frequent updates (e.g., theme, language preferences, user authentication state).
- Redux is better suited for large-scale applications with more complex state logic, frequent state updates, or where you need to manage data at a global level across many components. It offers more structure and scalability compared to Context.
Summary
- React Context: Simple, built into React, best for small to medium apps with minimal state sharing.
- Redux: Powerful, external library, best for larger applications with more complex state logic, where state needs to be shared and updated across many components.
Related Posts



